
I usually find it hard to open the browser and go to /bandwidth_distribution.sh to see who's using all the bandwidth, so I wrote bandwidth_distribution.sh.
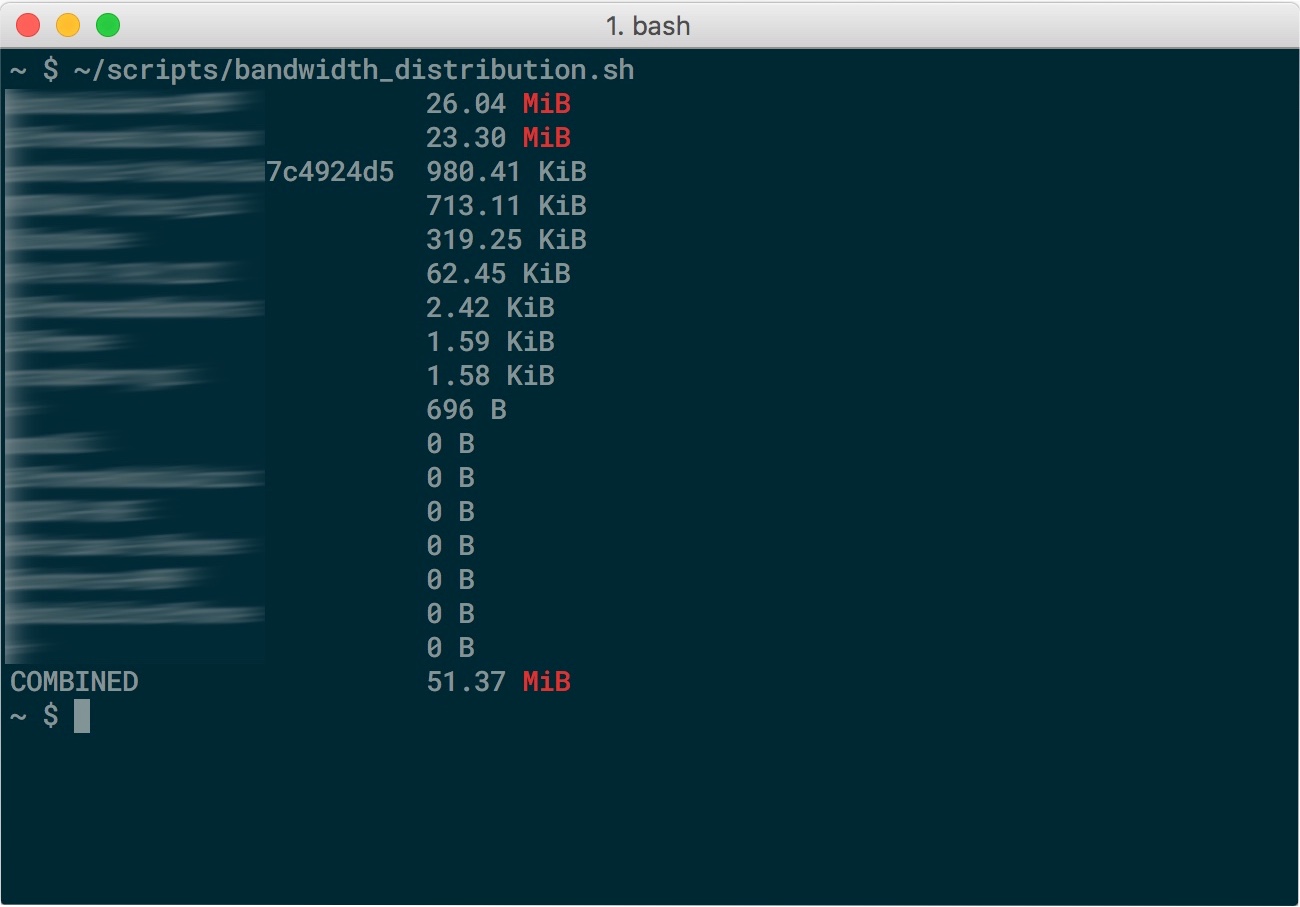
Code: Select all
#!/bin/bash
IP="192.168.1.1"
out=$(ssh root@${IP} "bw_get -i bdist1-download-minute-15 | awk 'FNR==NR{ a[\$3]=\$4; next } \$1 in a { \$1 = a[\$1] } { print }' /tmp/dhcp.leases -" | sort -gr -t' ' -k 2 | column -t | tr -s ' ')
# convert bytes to human readable form. e.g. 20456634 -> 19.51 MiB
bytesToHuman() {
local L_BYTES="${1:-0}"
local L_PAD="${2:-no}"
local L_BASE="${3:-1024}"
BYTESTOHUMAN_RESULT=$(awk -v bytes="${L_BYTES}" -v pad="${L_PAD}" -v base="${L_BASE}" 'function human(x, pad, base) {
if(base!=1024)base=1000
basesuf=(base==1024)?"iB":"B"
s="BKMGTEPYZ"
while (x>=base && length(s)>1)
{x/=base; s=substr(s,2)}
s=substr(s,1,1)
xf=(pad=="yes") ? ((s=="B")?"%5d ":"%8.2f") : ((s=="B")?"%d":"%.2f")
s=(s!="B") ? (s basesuf) : ((pad=="no") ? s : ((basesuf=="iB")?(s " "):(s " ")))
return sprintf( (xf " %s\n"), x, s)
}
BEGIN{print human(bytes, pad, base)}')
echo "$BYTESTOHUMAN_RESULT"
}
while read -r line; do
host=$(echo "$line" | cut -d' ' -f1)
bytes=$(echo "$line" | cut -d' ' -f2)
human=$(bytesToHuman "$bytes")
table+="$host,$human"
table+=$'\n'
done <<< "$out"
echo "$table" | column -s',' -t | sed "s,MiB,$(tput setaf 1)&$(tput sgr0)," | sed '1,1{H;1h;d;};$G'
You need SSH to be working, preferably using private key login AKA passwordless login, if you are experiencing slow SSH logins AKA it takes more than a second to connect, read my thread here, if you want to make connecting to your SSH even faster, Google SSH ControlMaster/ControlPersist.
Things to note
- This script meant to run on unix systems e.g. linux/macOS.
- If your router's IP is not 192.168.1.1, then change line 3.
- I'm not sure if this script will run on all Gargoyle installations.
- I'm sure there are so many ways to improve it.